Properties of RV
Contents
import torch
import matplotlib.pyplot as plt
import seaborn as sns
sns.reset_defaults()
#sns.set_context(context="talk", font_scale=1)
%matplotlib inline
%config InlineBackend.figure_format='retina'
import matplotlib as mpl
mpl.rcParams['axes.spines.right'] = False
mpl.rcParams['axes.spines.top'] = False
dist = torch.distributions
normal = dist.Normal(loc = 0., scale = 1.)
normal
Normal(loc: 0.0, scale: 1.0)
Properties of RV#
normal.mean
tensor(0.)
normal.loc
tensor(0.)
normal.scale
tensor(1.)
normal.stddev
tensor(1.)
normal.arg_constraints
{'loc': Real(), 'scale': GreaterThan(lower_bound=0.0)}
Drawing samples#
sample = normal.sample([1000])
sns.displot(sample)
<seaborn.axisgrid.FacetGrid at 0x15e71b5e0>
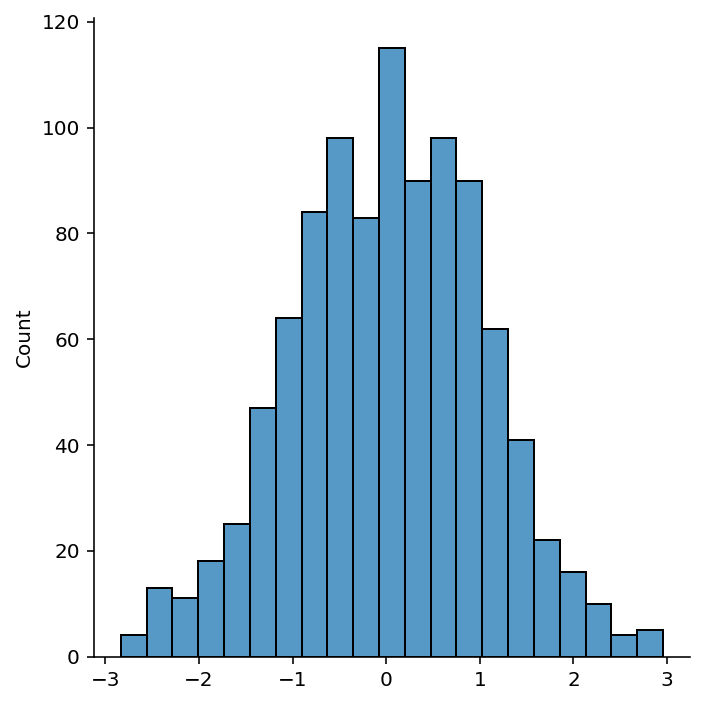
sns.displot(sample, kind='kde',bw_adjust=2, rug=True)
<seaborn.axisgrid.FacetGrid at 0x15ea847c0>
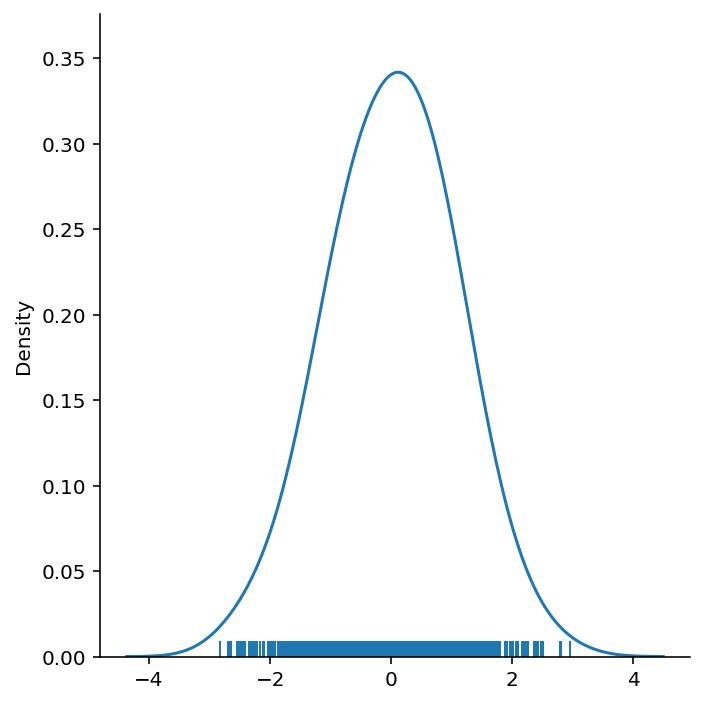
Finding pdf at a given point#
normal.log_prob(torch.tensor([1.]))
Plotting pdf over given range#
x = torch.linspace(-5., 5., 100)
pdf_x = normal.log_prob(x).exp()
plt.plot(x, pdf_x)
plt.xlabel("x")
plt.ylabel("PDF")
Text(0, 0.5, 'PDF')
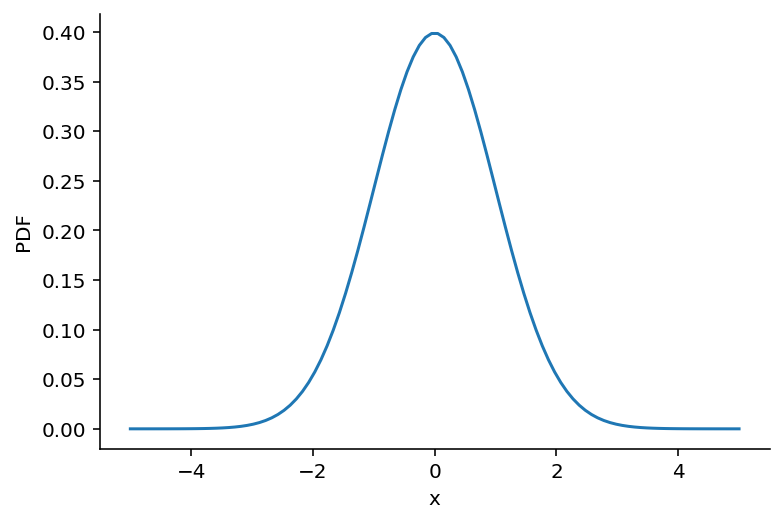
Finding cdf at a given point#
plt.plot(x, normal.cdf(x))
[<matplotlib.lines.Line2D at 0x15ed35670>]
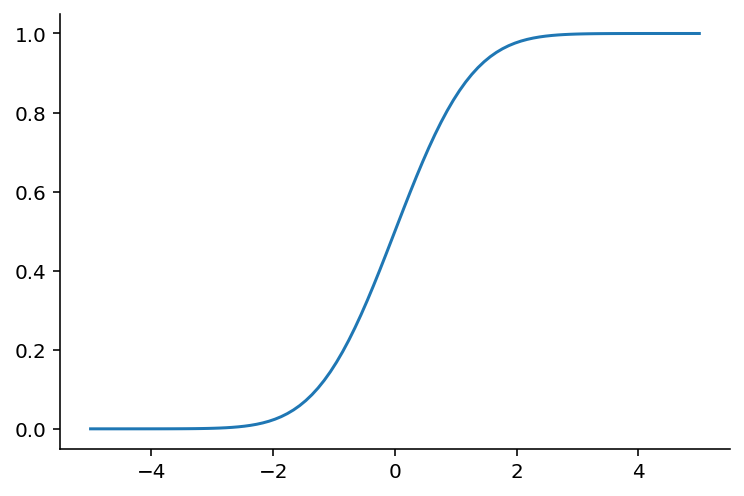
[x for x in dir(normal) if "__" not in x]
['_batch_shape',
'_event_shape',
'_extended_shape',
'_get_checked_instance',
'_log_normalizer',
'_mean_carrier_measure',
'_natural_params',
'_validate_args',
'_validate_sample',
'arg_constraints',
'batch_shape',
'cdf',
'entropy',
'enumerate_support',
'event_shape',
'expand',
'has_enumerate_support',
'has_rsample',
'icdf',
'loc',
'log_prob',
'mean',
'perplexity',
'rsample',
'sample',
'sample_n',
'scale',
'set_default_validate_args',
'stddev',
'support',
'variance']
normal.support
Real()
normal.entropy()
tensor(1.4189)
dist.Normal(1., 1).entropy()
tensor(1.4189)
dist.Normal(1., 2).entropy()
tensor(2.1121)
normal.perplexity()
tensor(4.1327)